Swift Day 2: Deep Diving Into Swift Syntax
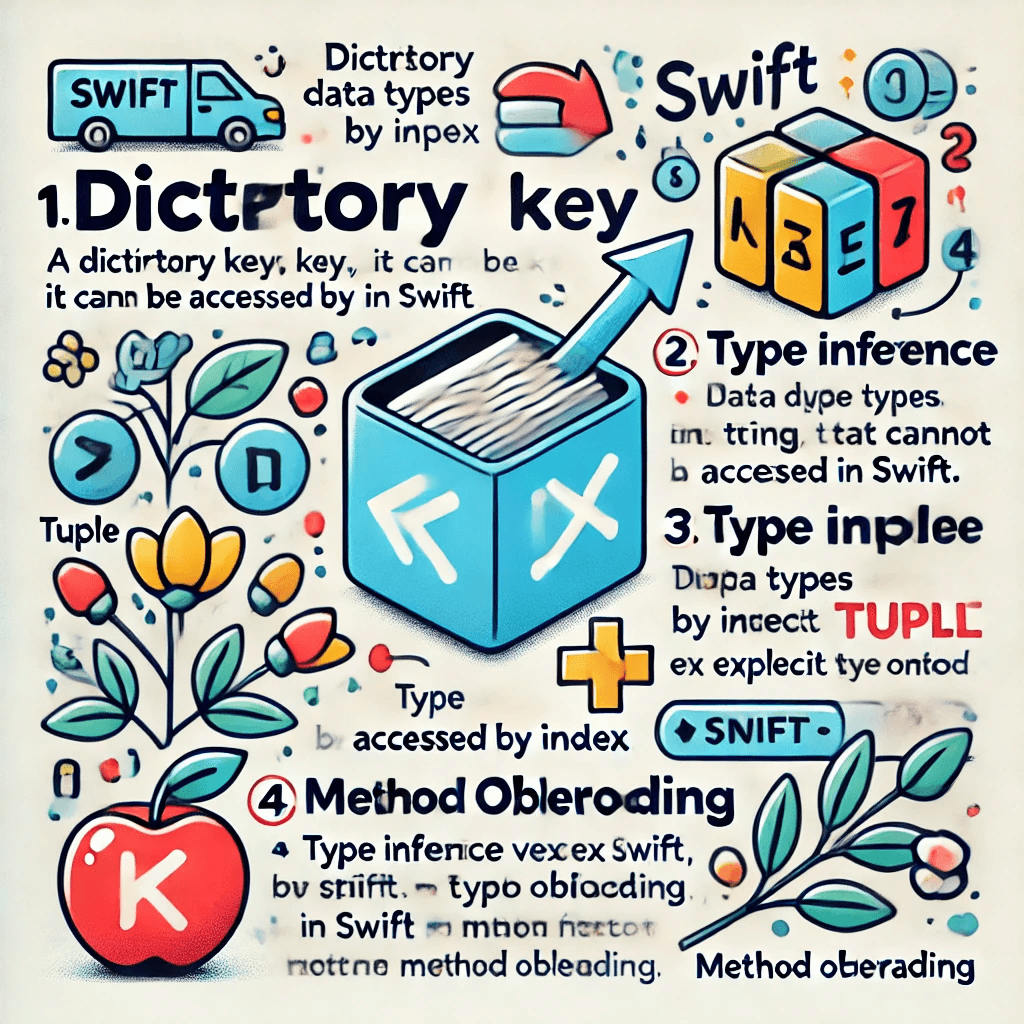
Day 2!
Hello everyone! Let's jump straight into day 2 of 100 days of Swift UI. I've noticed that a lot of the material covered in the course is simple/review, so I will be sharing interesting pieces from lessons 2-10 in todays blog post.
Dictionaries in Swift
One observation I made today is that Swift dictionaries are quite different from Java or Python dictionaries. In Swift, you cannot access keys by indexes.For example, take the following dictionary:
1swiftvar myDict = [ "light": "green", "street": "Westminster" ]
Trying to access the key street via the following line will result in a compilation error:
1swiftvar homeStreet = myDict[1]
This is because dictionaries are implementing the LazyMapCollection, which is not subscriptable with an int.This is ok though! Because in swift, searching dictionary by a key will always return an optional value. It is then up to the developer to handle the return value of the optional. If we would like to search the dictionary for a key, we can always use the contains() method.
Type Annotations Are Available
In Swift, type annotations are available but not always required. Majority of the time, we can use type inference for our variables. For me, the primary use case for type annotations will be to declare a variable without having to initialize it to a default value. Coming from the Java world, I enjoy using type annotations, especially for more complex types, as I can quickly visit their definitions and obtain context for the business logic I'm developing.
Tuples are of mixed type
In Swift, tuples can contain multiple types (Ints, Strings, Bools, etc). This is convenient as I was worried about the limitation of arrays and sets in Swift. Maybe I'm too used to Python and Javascript!
Overloading methods
In Swift, we can define functions with the same names but have different parameters/arguments, similar to overloaded methods in Java. For example:
1func hireEmployee(name: String) { }
2func hireEmployee(title: String) { }
3func hireEmployee(location: String) { }
the method hireEmployee
will perform different actions based on the parameter you pass. This is a form of self documentation and helpful for developing larger projects.