Swift Day 1: Swift Strings versus Java Strings
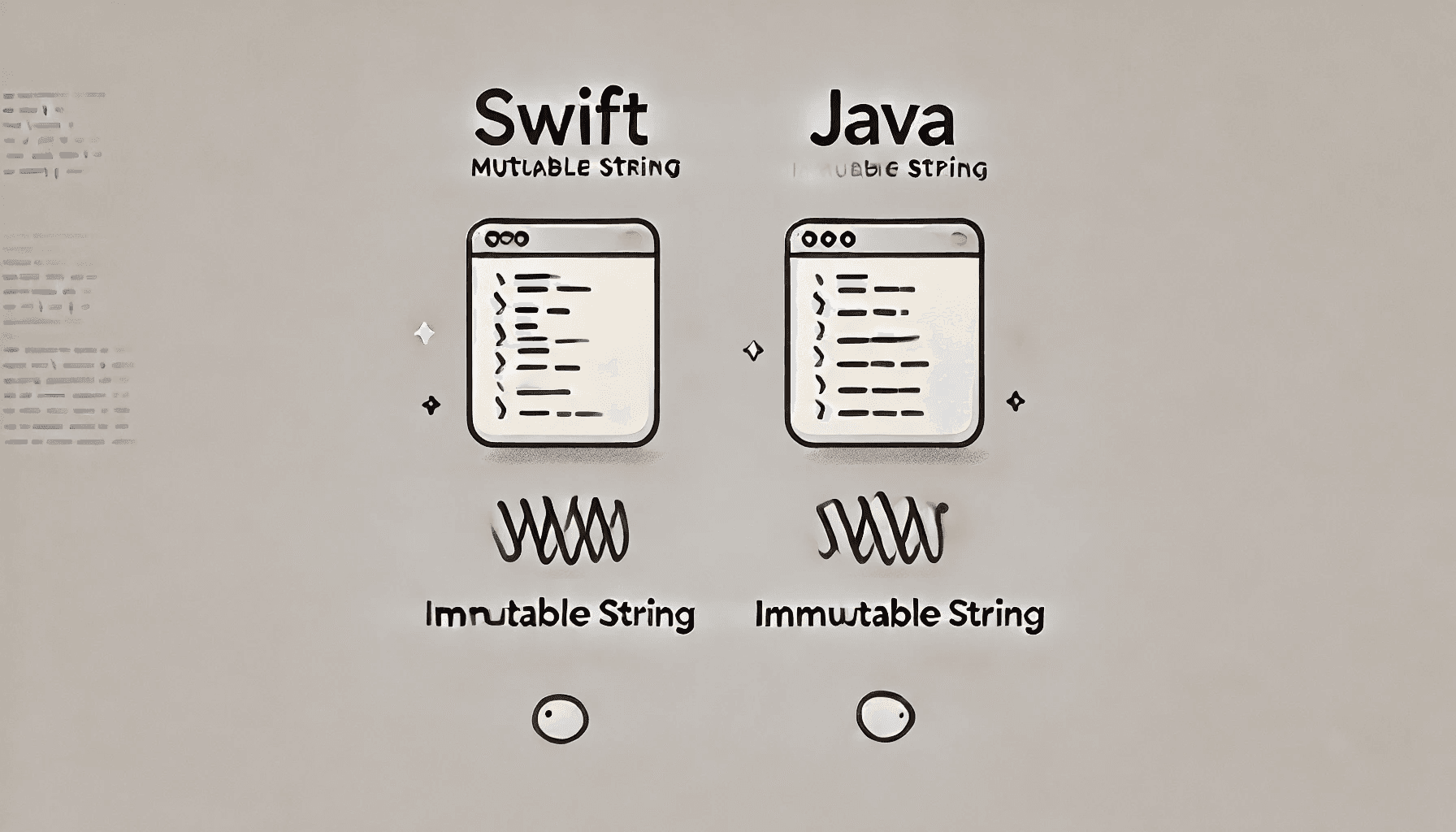
Background
My primary language of development in my day job is Java. Java is great! I feel safe when I use a strongly typed language, and IntelliJ makes me feel happy when I write code and it doesn't crash my M2 Pro (this only happens once a week!)
Swift String is mutable
Today, I learned something new about Swift that makes me feel a little less fond of Java, and that is the Swift String. Since Swift Strings are mutable. For example (sorry the file says Python I need to update my CMS):
1var someString = "This is" // line 1
2someString += "the same string." // line 2
someString is still the same string in line 1, but it is simply modified. If we would like for the String to be immutable, we simply set the String to be a constant:
1let constString = "we can't change this string"
Java String is Immutable
In Java, String classes are immutable. Whenever we would like to make a new String via concatenation, we create an entirely new String in memory. For example:
1String someString = "This is"; // line 1
2someString += "a new string."; // line 2
someString is actually two different strings between line 1 and line 2. They only appear to be the same String due to reference reassignment.
Why This (Might) Matter
Modifying existing strings in memory (safely that is) is more performant than creating new copies of a string. In theory, if you were performing a considerable amount of String concatenation/manipulation, you could perform the same task more quickly in Swift than in Java. This is a small subset of teams, but if you are on a full stack iOS team, it could be more beneficial to write a high volume string parsing micro service in Swift instead of Java.